When you look at a chaotic crowd, have you ever wondered how some people can spot order in the chaos? That’s what a Support Vector Machine (SVM) does in the world of data. It’s a Machine Learning model that’s brilliant at separating data into distinct groups, even when things seem impossibly tangled.
In this article, we’ll explore how SVM works its magic to classify data, tackle tough scenarios like outliers and non-linearity, and why it’s often the go-to tool for small, complex datasets. Let’s dive in!

What Is Classification?
Before we get into Support Vector Machine, let’s first understand classification. Imagine you’re organizing books in a library. Some belong in the “fiction” section, and others go into “non-fiction.” This task of sorting is what machine learning calls classification—grouping data into categories.
For example:
- Predicting if it will rain tomorrow? That’s classification.
- Estimating how much rain will fall? That’s regression.
Classification algorithms focus on dividing data into groups, often called classes. Models like Decision Trees create rules for sorting, while others like K-Nearest Neighbors (KNN) look at how similar one data point is to another.
SVM, however, takes a unique approach—it separates data by finding the “perfect” boundary.
How Does Support Vector Machine Work?
SVM is all about finding the optimal hyperplane. But what does that mean? Let’s break it down:
Step 1: Visualizing the Data
Imagine you have two groups of points on a graph. These points could represent customers who bought a product (Group A) and those who didn’t (Group B). Your goal is to draw a line that separates these two groups.
If the data is in two dimensions, this “line” does the job. But if the data exists in more dimensions (like age, income, and purchase history), this separating line becomes a plane or a hyperplane.
How SVM Finds the Best Hyperplane
Not all lines are created equal. Some lines might separate the groups but leave very little space between the line and the points. That’s where Support Vector Machine shines—it looks for the line (or hyperplane) that creates the widest possible gap between the two groups.
The Key Terms You Should Know:
- Margin: The gap between the hyperplane and the nearest data points from each group.
- Support Vectors: The data points closest to the hyperplane. These are critical in defining the boundary.
SVM’s goal is to maximize the margin. Why? Because a larger margin reduces the chance of misclassifying new data points.
Think of it as walking a tightrope. The wider the net below, the safer you are.
What About Outliers?
Outliers are tricky. They’re like that one friend who shows up to a formal party in flip-flops—completely out of place but impossible to ignore.
If an outlier becomes a support vector, it can distort the hyperplane and lead to poor predictions. Support Vector Machine has a clever solution: soft margins.
What Are Soft Margins?
Soft margins allow SVM to tolerate a bit of misclassification. This trade-off between bias and variance ensures that the model doesn’t overreact to outliers, resulting in better predictions for new data.
What If the Data Can’t Be Separated Linearly?
Let’s face it—real-world data isn’t always simple. Sometimes, no straight line can divide the groups. This is where Support Vector Machine kernels come into play.
What Is a Kernel?
A kernel is like a magician’s trick—it transforms data into a higher-dimensional space where it becomes linearly separable.
Imagine trying to separate two spirals on a flat surface. Impossible, right? Now imagine lifting one spiral off the surface into 3D space. Suddenly, separating them is a breeze.
Popular kernels include:
- Linear Kernel: For data that’s already linearly separable.
- Polynomial Kernel: Adds complexity to capture intricate patterns.
- Radial Basis Function (RBF) Kernel: Ideal for very complex, nonlinear data.
What Are the Advantages and Disadvantages of SVM in Machine Learning?
Like any tool, Support Vector Machine has its pros and cons. Here’s a quick overview:
Advantages:
- Effective in high-dimensional spaces: SVM works well when there are many features.
- Versatile: It supports different kernel functions, making it adaptable to various data types.
- Robust to outliers: Soft margins help balance precision and flexibility.
Disadvantages:
- Computationally intensive: Training SVM can be slow for large datasets.
- Not ideal for overlapping classes: SVM struggles when the margin is hard to define.
- Sensitive to hyperparameters: Proper tuning is essential for optimal performance.
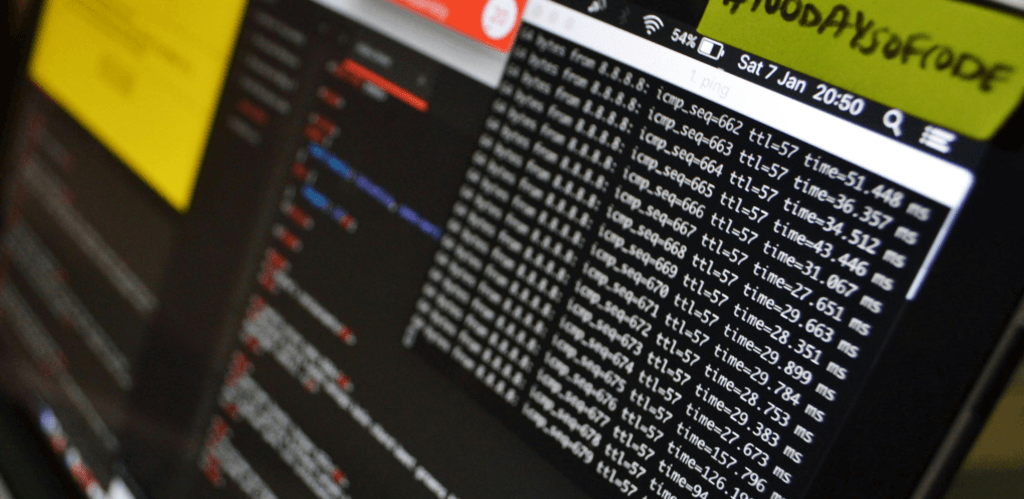
Support Vector Machine Hyperparameters
SVM’s performance depends heavily on its hyperparameters. Key ones include:
- C (Regularization Parameter): Controls the trade-off between achieving a large margin and minimizing misclassification. Higher values aim for perfect classification, while lower values allow more slack.
- Kernel Type: Determines the decision boundary. Options include linear, polynomial, and RBF kernels.
- Gamma: Defines how far the influence of a single data point reaches. A higher gamma focuses on nearby points, while a lower gamma considers broader trends.
Support Vector Machine Applications
SVM is used across various domains to solve classification and regression problems. Here are a few examples:
- Text Categorization: Sorting emails into spam or non-spam.
- Image Recognition: Identifying objects in photos or videos.
- Bioinformatics: Classifying genes or predicting diseases.
- Finance: Detecting fraudulent transactions.
Support Vector Regression
While Support Vector Machine is primarily known for classification, it can also handle regression tasks. This is called Support Vector Regression (SVR). Instead of finding a hyperplane that separates classes, SVR finds one that predicts continuous values within a margin of tolerance.
SVR is great for applications like predicting housing prices, stock values, or weather conditions.
Quantum Support Vector Machine
In the era of Quantum Computing, researchers have explored Quantum SVM (QSVM). This approach leverages quantum algorithms to process data faster, making SVM scalable for extremely large datasets. QSVM is still in its infancy but holds promise for fields like cryptography and big data analytics.
Multiclass SVM
While Support Vector Machine naturally handles binary classification, it can also tackle problems with multiple classes using techniques like:
- One-vs-One: Breaks the problem into multiple binary classifications for each pair of classes.
- One-vs-Rest: Builds one classifier for each class versus the rest.
These approaches enable SVM to classify datasets with more than two categories.
Support Vector Machine Example
Let’s consider an example of using Support Vector Machine for spam email detection:
- Data Collection: Gather labeled emails (spam or not spam).
- Feature Extraction: Convert email content into numerical features using techniques like TF-IDF.
- Training: Train an SVM model with an appropriate kernel.
- Prediction: Use the trained model to classify new emails.
Here’s a simplified code snippet:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.svm import SVC
from sklearn.model_selection import train_test_split
# Example data
emails = [“Buy now!”, “Meeting at 3 PM”, “Limited offer!”]
labels = [1, 0, 1] # 1 = Spam, 0 = Not Spam
# Feature extraction
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(emails)
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(X, labels, test_size=0.2)
# Train SVM
model = SVC(kernel=’linear’)
model.fit(X_train, y_train)
# Predict
predictions = model.predict(X_test)
print(“Predictions:”, predictions)
Final Thoughts
Support Vector Machine (SVM) may seem complex at first, but they’re incredibly powerful when you understand their workings. Whether you’re dealing with messy data, outliers, or nonlinear patterns, SVM can help you find order in the chaos.
So, the next time you’re wrestling with a classification problem, give SVM a try. It just might be the pro-level tool you need to separate your data with precision and flair.
Thank you for reading! I would love to hear your thoughts and feedback in the comments section below.
Ready to dive deeper? Check out these resources:
- Linear Regression Algorithm Simplified: The Ultimate Backbone of Predictive Modeling
- How Artificial Intelligence is Changing the Future of Work, Life, and Innovation in Extraordinary Ways
- Neural Networks 101: Build the Brilliant Brain of a Machine
- Random Forest Algorithm Decoded: The Power of Multiple Trees in Machine Learning
- Supervised vs Unsupervised Learning: The Ultimate Guide to Understanding the Difference
- Reinforcement Learning Models: Innovative Algorithms That Evolve and Excel Through Trial and Error
- Logistic Regression vs Linear Regression: Discover the Key Differences and When to Choose Each